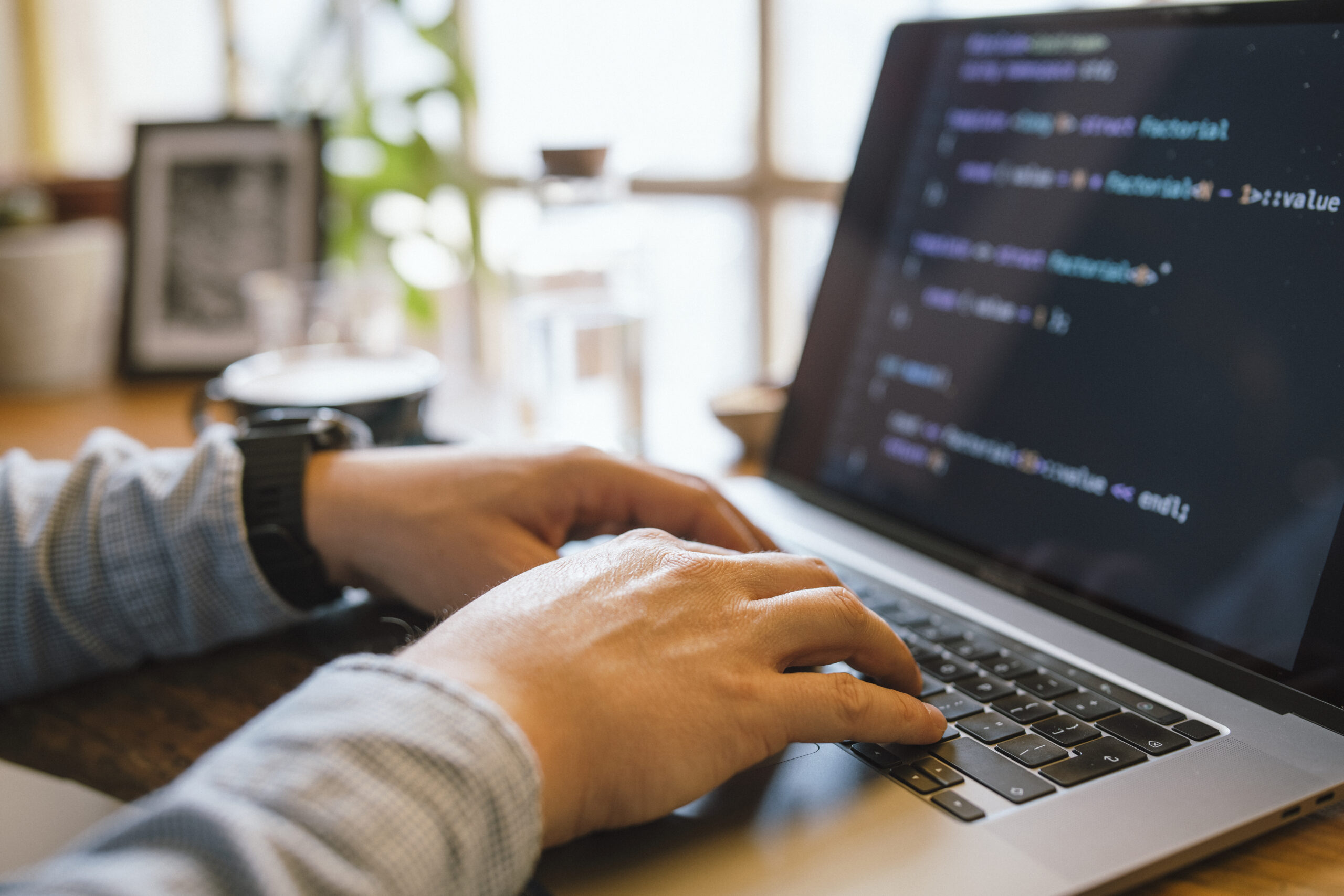
Debugging is The most important — nevertheless generally missed — abilities within a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Studying to Believe methodically to solve issues effectively. Regardless of whether you're a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and considerably improve your efficiency. Here i will discuss quite a few procedures that can help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
One of the fastest strategies developers can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is a person Component of enhancement, figuring out the way to interact with it effectively all through execution is equally significant. Modern day improvement environments appear Outfitted with potent debugging abilities — but many builders only scratch the surface area of what these tools can perform.
Get, for instance, an Built-in Development Natural environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment let you established breakpoints, inspect the worth of variables at runtime, action by way of code line by line, and also modify code on the fly. When applied properly, they Permit you to notice precisely how your code behaves all through execution, that's a must have for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for front-conclude builders. They let you inspect the DOM, observe network requests, watch true-time performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can change disheartening UI problems into workable duties.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep Management over operating processes and memory administration. Learning these resources could have a steeper Studying curve but pays off when debugging functionality difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn out to be relaxed with Model Command techniques like Git to be aware of code record, find the exact moment bugs had been released, and isolate problematic variations.
Ultimately, mastering your tools indicates heading over and above default options and shortcuts — it’s about acquiring an personal expertise in your development atmosphere to ensure that when concerns come up, you’re not missing in the dead of night. The greater you already know your instruments, the greater time it is possible to commit resolving the particular challenge in lieu of fumbling by the method.
Reproduce the challenge
The most significant — and infrequently forgotten — methods in successful debugging is reproducing the trouble. Prior to jumping in to the code or making guesses, builders need to have to make a constant environment or state of affairs where the bug reliably seems. With no reproducibility, fixing a bug results in being a video game of possibility, frequently leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is collecting just as much context as is possible. Check with queries like: What actions brought about the issue? Which ecosystem was it in — growth, staging, or production? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it becomes to isolate the exact conditions less than which the bug happens.
Once you’ve gathered enough facts, attempt to recreate the condition in your neighborhood environment. This might mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If the issue appears intermittently, take into consideration composing automatic tests that replicate the edge scenarios or condition transitions associated. These tests not merely help expose the trouble and also prevent regressions Down the road.
Occasionally, The problem can be environment-certain — it'd materialize only on particular functioning systems, browsers, or beneath unique configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a move — it’s a state of mind. It needs endurance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. Having a reproducible situation, You can utilize your debugging equipment far more properly, take a look at probable fixes safely and securely, and communicate more clearly with your team or users. It turns an summary criticism right into a concrete obstacle — Which’s the place developers thrive.
Study and Comprehend the Error Messages
Mistake messages tend to be the most useful clues a developer has when a thing goes Completely wrong. Rather then seeing them as disheartening interruptions, builders should really study to deal with error messages as immediate communications within the technique. They usually tell you just what happened, where it took place, and often even why it occurred — if you know the way to interpret them.
Start out by reading through the concept very carefully As well as in entire. Several builders, especially when less than time force, look at the very first line and straight away start off creating assumptions. But further inside the mistake stack or logs may possibly lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and understand them initially.
Break the error down into areas. Is it a syntax error, a runtime exception, or a logic error? Will it level to a selected file and line quantity? What module or perform activated it? These concerns can tutorial your investigation and stage you towards the liable code.
It’s also beneficial to be familiar with the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and learning to recognize these can considerably accelerate your debugging system.
Some mistakes are obscure or generic, As well as in those situations, it’s very important to examine the context during which the mistake occurred. Examine linked log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings both. These generally precede larger problems and provide hints about likely bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them the right way turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra economical and confident developer.
Use Logging Properly
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When used effectively, it provides real-time insights into how an software behaves, aiding you realize what’s taking place under the hood without having to pause execution or move in the code line by line.
A very good logging system starts off with understanding what to log and at what level. Typical logging levels include DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic data for the duration of advancement, Information for general events (like successful get started-ups), Alert for prospective troubles that don’t split the application, Mistake for true issues, and FATAL if the program can’t carry on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your process. Target important situations, point out alterations, input/output values, and important selection points with your code.
Format your log messages Evidently and constantly. Contain context, such as timestamps, ask for IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Specifically valuable in creation environments where by stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about equilibrium and clarity. Using a very well-imagined-out logging solution, you'll be able to lessen the time it takes to spot troubles, attain deeper visibility into your programs, and Enhance the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To proficiently identify and resolve bugs, builders ought to solution the process like a detective solving a mystery. This frame of mind helps break down complicated concerns into workable sections and observe clues logically to uncover the foundation cause.
Start by gathering evidence. Consider the indicators of the situation: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, obtain just as much suitable info as you are able to with out jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent photo of what’s going on.
Upcoming, sort hypotheses. Question by yourself: What may be leading to this conduct? Have any changes recently been built towards the codebase? Has this issue happened ahead of below equivalent situations? The objective is to slender down options and discover potential culprits.
Then, take a look at your theories systematically. Try and recreate the trouble in a managed surroundings. In the event you suspect a selected operate or component, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcomes guide you closer to the reality.
Shell out close consideration to little details. Bugs generally conceal during the minimum envisioned areas—similar to a missing semicolon, an off-by-a person mistake, or perhaps a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of completely understanding it. Momentary fixes might disguise the actual difficulty, just for it to resurface later.
And lastly, preserve notes on Anything you attempted and learned. Just as detectives log their investigations, documenting your debugging system can conserve time for long term difficulties and assist Some others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical capabilities, solution difficulties methodically, and develop into more effective at uncovering concealed issues in sophisticated devices.
Create Exams
Writing exams is one of the best tips on how to boost your debugging abilities and All round growth performance. Tests not only support capture bugs early and also function a security Web that offers you self-assurance when producing alterations on your codebase. A very well-analyzed software is much easier to debug as it means that you can pinpoint accurately where by and when a dilemma occurs.
Start with unit checks, which focus on specific capabilities or modules. These smaller, isolated assessments can speedily reveal no matter whether a particular bit of logic is Performing as predicted. Each time a examination fails, you right away know exactly where to appear, significantly reducing some time expended debugging. Unit assessments are Specially beneficial for catching regression bugs—problems that reappear after Beforehand currently being mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These help make sure several areas of your application get the job done collectively smoothly. They’re significantly valuable for catching bugs that happen in complex devices with numerous elements or services interacting. If anything breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely think critically regarding your code. To test a feature appropriately, you need to be aware of its inputs, expected outputs, and edge scenarios. This degree of knowledge Normally potential customers to better code framework and much less bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails persistently, you can give attention to correcting the bug and watch your examination go when the issue is solved. This solution ensures that the identical bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing sport into a structured and predictable course of action—helping you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to be immersed in the issue—looking at your display for hrs, striving Option following Remedy. But Among the most underrated debugging instruments is solely stepping absent. Having breaks allows you reset your intellect, cut down frustration, and often see The difficulty from the new standpoint.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain results in being a lot less successful at dilemma-fixing. A short wander, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your target. Several developers report locating the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist reduce burnout, In particular for the duration of for a longer time debugging classes. Sitting down before a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed energy and also a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to move all-around, stretch, or do a little something unrelated to code. It may well truly feel counterintuitive, Primarily below limited deadlines, however it essentially leads to more rapidly and more practical debugging Over time.
To put it briefly, using breaks will not be a sign of weak point—it’s a sensible strategy. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every single bug you experience is much more than simply A short lived setback—it's an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you something useful in case you make the effort to replicate and examine what went Mistaken.
Start out by inquiring you a few important queries after the bug is settled: What induced it? Why did it go unnoticed? Could it are caught previously with superior techniques like device screening, code opinions, or logging? The solutions often reveal blind places in the workflow or being familiar with and help you build much better coding patterns going ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you acquired. Eventually, you’ll begin to see designs—recurring concerns or typical mistakes—you could proactively prevent.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be Primarily highly effective. No matter whether it’s through a Slack information, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical portions of your advancement journey. After all, several of the very best builders are not those who write best code, but those who continually learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So next time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, much more able read more developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and tolerance — however the payoff is big. It will make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become superior at Anything you do.